js 异步…
eventLoop
Event Loop 执行顺序图:
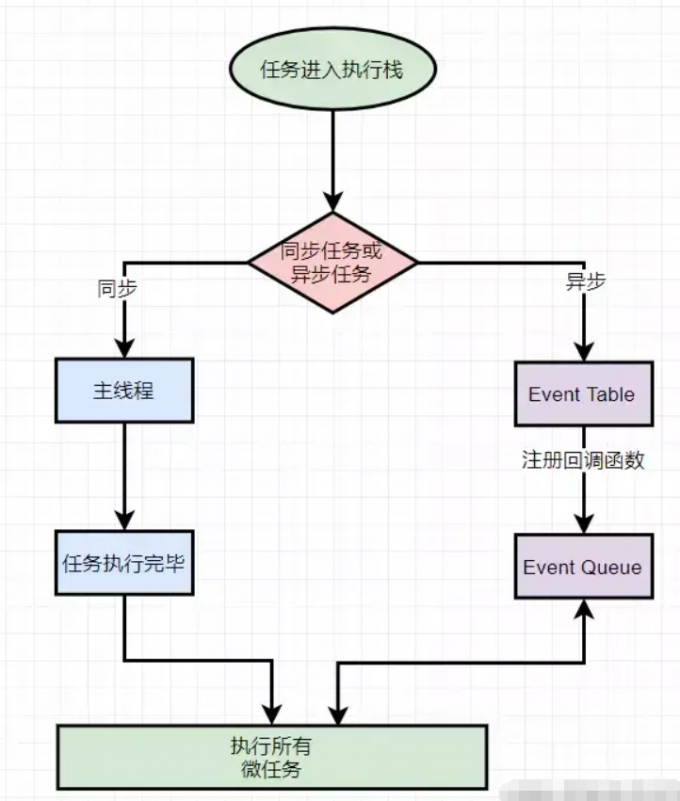
异步代码常见规范
- reject 一个 Error 对象
1 2 3 4 5
| Promise.reject("error reason");
Promise.reject(new Error("error reason"));
|
- 不要在 Promise 上使用 async
不要把 async 函数传递给 Promise 构造函数,因为没有必要,其次如果 async 函数异常,那么你的 promise 并不会 reject
1 2 3 4 5 6
| new Promise(async (resolve, reject) => {})
new Promise((resolve, reject) => { async function(){coding....}()})
|
- 不要使用 await 在循环中
尽可能将这写异步的任务改为并发,可以大幅提高代码执行效率
1 2 3 4 5 6 7 8 9 10 11 12
| for (const apiPath of paths) { const { data } = await request(apiPath); }
const results = []; for (const apiPath of paths) { const res = resquest(apiPath); results.push(res); } await Promise.all(results);
|
- 不要再 Promise 中使用 return 语句
Promise 构造函数不希望回调返回任何形式的值
return 一般用来控制该函数中的执行流程
1 2 3 4 5
| new Promise((resolve, reject) => { return resolve(1); console.log("2"); });
|
1 2 3 4 5 6 7 8 9 10
| new Promise((resolve, reject) => { if (isOK) return "ok"; return "not ok"; });
new Promise((resolve, reject) => { if (isOK) resolve("ok"); reject(new Error("not ok")); });
|
- 别忘了异常处理
1 2 3 4 5 6 7
| asyncPromise().then(() => {});
asyncPromise() .then(() => {}) .catch(() => {});
|
1 2 3 4 5 6 7 8 9 10
| const result = await asyncPromise()
try { const result = await asyncPrmise() } catch() { }
|